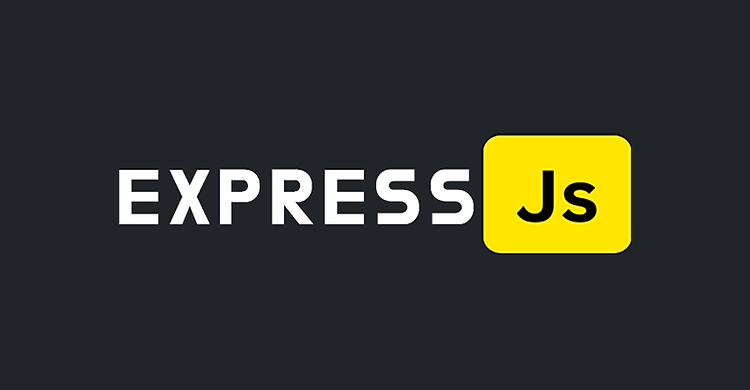
๐ ๊ฐ๋ฐ ํ๊ฒฝ
๐พ Visual Studio Code
Visual Studio Code - Code Editing. Redefined
Visual Studio Code is a code editor redefined and optimized for building and debugging modern web and cloud applications. Visual Studio Code is free and available on your favorite platform - Linux, macOS, and Windows.
code.visualstudio.com
๐พ MySQL Workbench
MySQL :: Download MySQL Workbench
Select Operating System: Select Operating System… Microsoft Windows Ubuntu Linux Red Hat Enterprise Linux / Oracle Linux Fedora macOS Source Code Select OS Version: All Windows (x86, 64-bit) Recommended Download: Other Downloads: Windows (x86, 64-bit), M
dev.mysql.com
๐พ Node.js
Node.js — Run JavaScript Everywhere
Node.js® is a JavaScript runtime built on Chrome's V8 JavaScript engine.
nodejs.org
๐พ Express.js
Express.js ์ค์น ๋ช ๋ น์ด
npm install express
๐พ MySQL2
MySQL2 ์ค์น ๋ช ๋ น์ด
npm install mysql2
๐พ Nodemon
Nodemon ์ค์น ๋ช ๋ น์ด
npm install nodemon
๐พ Nunjucks
Nunjucks ์ค์น ๋ช ๋ น์ด
npm install nunjucks
1. MySQL Database ์ค์
MySQL Workbench์์ ๋ฐ์ดํฐ๋ฒ ์ด์ค์ ํ ์ด๋ธ์ ๋ง๋ ๋ค.
์์๋ก 'nodejs' ์คํค๋ง์ member ํ
์ด๋ธ์ ๋ง๋ค์๋ค.
CREATE TABLE `nodejs`.`member` (
`id` VARCHAR(50) NULL,
`pw` VARCHAR(50) NULL,
`nick` VARCHAR(50) NULL);
2. Express.js ๊ธฐ๋ณธ ํด๋ ์์ฑ
ํด๋น ํ๋ก์ ํธ ์๋์ ๋ค์๊ณผ ๊ฐ์ ํด๋๋ค์ ์์ฑํ๋ค.
๐ config : ์ค์ ์ ๊ด๋ จ๋ ์ ๋ณด๋ค์ ์ ์ฅ (DB ์ฐ๊ฒฐ ์ ๋ณด, API KEY ๋ฑ)
๐ public : ์ ์ ์ธ ํ์ผ๋ค์ ๊ด๋ฆฌํ๋ ๊ณต๊ฐ (CSS, JS, IMG, VIDEO ๋ฑ)
๐ routes : ๊ฒฝ๋ก๋ฅผ ๊ด๋ฆฌํ๋ ๊ณต๊ฐ
๐ views : ๋์ ์ธ ์นํ์ด์ง๋ฅผ ๊ด๋ฆฌํ๋ ๊ณต๊ฐ
3. Node.js / Exress.js์ MySQL DB ์ฐ๋
์๋ ์์๋ ํ์๊ฐ์ ๊ณผ ๋ก๊ทธ์ธ์ํ๋ ๊ณผ์ ์ด๋ค.
1) app.js
๐ project > ๐ app.js
const express = require("express");
const app = express();
const bodyParser = require("body-parser");
const nunjucks = require("nunjucks");
const mainRouter = require("./routes/mainRouter");
const userRouter = require("./routes/userRouter");
app.use(bodyParser.urlencoded({ extended: true }));
app.set("view engine", "html");
nunjucks.configure("views", {
express: app,
watch: true,
});
app.use("/", mainRouter);
app.use("/user", userRouter);
app.listen(3000);
2) db.js
DB์ ๊ด๋ จ๋ ์ ๋ณด๋ config ํด๋ ์๋์ db.js์ ์์ฑํ๋ค.
๐ config > ๐ db.js
// mysql2 ๋ชจ๋ ๋ถ๋ฌ์ค๊ธฐ
const mysql = require("mysql2");
// DB ์ฐ๊ฒฐ ์ ๋ณด
const conn = mysql.createConnection({
host: "localhost",
port: 3306,
user: "root",
password: "1234",
database: "nodejs",
});
// ์ฐ๊ฒฐ ์์
conn.connect();
console.log("DB ์ฐ๊ฒฐ ์ฑ๊ณต!");
module.exports = conn;
3) html
๐ views > ๐ main.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=<device-width>, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<h1>ํ์๊ด๋ฆฌ ์์คํ
</h1>
<a href="join">ํ์๊ฐ์
</a>
<a href="login">๋ก๊ทธ์ธ</a>
</body>
</html>
๐ views > ๐ join.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<h1>ํ์๊ฐ์
ํ์ด์ง</h1>
<form action="http://localhost:3000/user/join" method="post">
id : <input type="text" name="id" /> <br />
pw : <input type="password" name="pw" /> <br />
nick : <input type="text" name="nick" /> <br />
<input type="submit" />
</form>
</body>
</html>
๐ views > ๐ login.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<h1>๋ก๊ทธ์ธ ํ์ด์ง</h1>
<form action="http://localhost:3000/user/login" method="post">
id : <input type="text" name="id" /> <br />
pw : <input type="password" name="pw" /> <br />
<input type="submit" />
</form>
</body>
</html>
4) router.js
๐ routes > ๐ mainRouter.js
const express = require("express");
const router = express.Router();
// 1. ๋ฉ์ธ
router.get("/", (req, res) => {
res.render("main");
});
// 2. ํ์๊ฐ์
router.get("/join", (req, res) => {
res.render("join");
});
// 3. ๋ก๊ทธ์ธ
router.get("/login", (req, res) => {
res.render("login");
});
module.exports = router;
๐ routes > ๐ userRouter.js
const express = require("express");
const router = express.Router();
const conn = require("../config/db");
// 1. ํ์๊ฐ์
router.post("/join", (req, res) => {
let { id, pw, nick } = req.body;
// DB์ ์ฐ๊ฒฐํด์ ๋ฐ์ดํฐ ์ฒ๋ฆฌ
// 1) ์ฒ๋ฆฌํ sql๋ฌธ ์์ฑ
// 2) ์
๋ ฅํ ๋ฐ์ดํฐ๊ฐ ์๋ ๊ฒฝ์ฐ์ ๋งค๊ฐ๋ณ์์ ๊ฐ์ ๋ฃ์ด์ฃผ๊ธฐ
// 3) ๋ฐ์ดํฐ๋ฅผ ์ฒ๋ฆฌํ ์ฝ๋ฐฑํจ์ ์ ์
let sql = "insert into member values(?, ?, ?)";
conn.query(sql, [id, pw, nick], (err, rows) => {
console.log("ํ์๊ฐ์
DB ๊ฒฐ๊ณผ : ", rows);
// ๊ฒฐ๊ณผ๊ฐ์ด ์๋ ๊ฒฝ์ฐ
if (rows) {
res.redirect("/");
}
});
});
// 2. ๋ก๊ทธ์ธ
router.post("/login", (req, res) => {
let { id, pw } = req.body;
// DB์ ์ฐ๊ฒฐํด์ ๋ฐ์ดํฐ ์ฒ๋ฆฌ
// 1) ์ฒ๋ฆฌํ sql๋ฌธ ์์ฑ
// 2) ์
๋ ฅํ ๋ฐ์ดํฐ๊ฐ ์๋ ๊ฒฝ์ฐ์ ๋งค๊ฐ๋ณ์์ ๊ฐ์ ๋ฃ์ด์ฃผ๊ธฐ
// 3) ๋ฐ์ดํฐ๋ฅผ ์ฒ๋ฆฌํ ์ฝ๋ฐฑํจ์ ์ ์
let sql = "select * from member where id = ? and pw = ?";
conn.query(sql, [id, pw], (err, rows) => {
console.log("๋ก๊ทธ์ธ DB ๊ฒฐ๊ณผ : ", rows);
// select๋ฌธ์ ์ฌ์ฉ ์ ์ฃผ์์
// 1) Data๋ฅผ ๋ฐํํ ๋ ๋ฐฐ์ด ํํ๋ก ์ ๊ณต
// 2) ์กฐํ ๊ฒฐ๊ณผ๊ฐ ์๋๋ผ๋ ๋น์ด์๋ ๋ฐฐ์ด์ ๋ฐํํ๋ค.
// 3) ์กฐ๊ฑด์ ๋น๊ตํ ๋ ๋ฐฐ์ด์ ๊ธธ์ด๋ฅผ ๊ฐ์ง๊ณ ํ์ธํ๋ค.
// rows > 0๋ก ๋น๊ตํ๋ฉด ์๋๋ ์ด์ : ๋ฐฐ์ด๊ณผ ์ซ์๋ฅผ ๋น๊ตํ๋ฉด ํญ์ false์ด๋ค.
if (rows.length > 0) {
console.log("๋ก๊ทธ์ธ ์ฑ๊ณต");
res.redirect("/");
} else {
console.log("๋ก๊ทธ์ธ ์คํจ");
}
});
});
module.exports = router;
4. ์์ฝ ์ ๋ฆฌ
Express.js ์ค์น -> Express ๊ธฐ๋ณธ ํด๋ ์์ฑ -> app.js ์์ฑ -> db.js ์์ฑ -> html ์์ฑ -> router ์์ฑ
* ์ถ๊ฐ๋ก MySQL2์ ์ค์นํ์ฌ ๋ฐ์ดํฐ๋ฒ ์ด์ค ์ฐ๊ฒฐ, Nodemon์ ์ค์นํ์ฌ ์๋ ์๋ฒ ์ฌ์์ ๊ธฐ๋ฅ ๊ตฌํ, , Nunjucks๋ฅผ ์ค์นํ์ฌ ํ ํ๋ฆฟ ์์ง์ผ๋ก ๋์ HTML ํ์ด์ง ์์ฑ ๋ฑ ๋ค์ํ ๋ผ์ด๋ธ๋ฌ๋ฆฌ๋ฅผ ์ค์นํ์ฌ ํ๋ก์ ํธ์ ๊ธฐ๋ฅ์ฑ์ ํ์ฅํ๊ณ ๊ฐ๋ฐ ํธ์์ฑ์ ๋์ผ ์ ์๋ค.
'Framework > Express' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
Node.js / Express.js์์ Router ์กฐ์ํ๊ธฐ (0) | 2024.07.28 |
---|---|
Node.js / Express์์ GET๊ณผ POST ์์ฒญ ์ฒ๋ฆฌํ๋ ๋ฐฉ๋ฒ (0) | 2024.07.22 |
ํฌ์คํ ์ด ์ข์๋ค๋ฉด "์ข์์โค๏ธ" ๋๋ "๊ตฌ๋ ๐๐ป" ํด์ฃผ์ธ์!